Schedule To C Definition
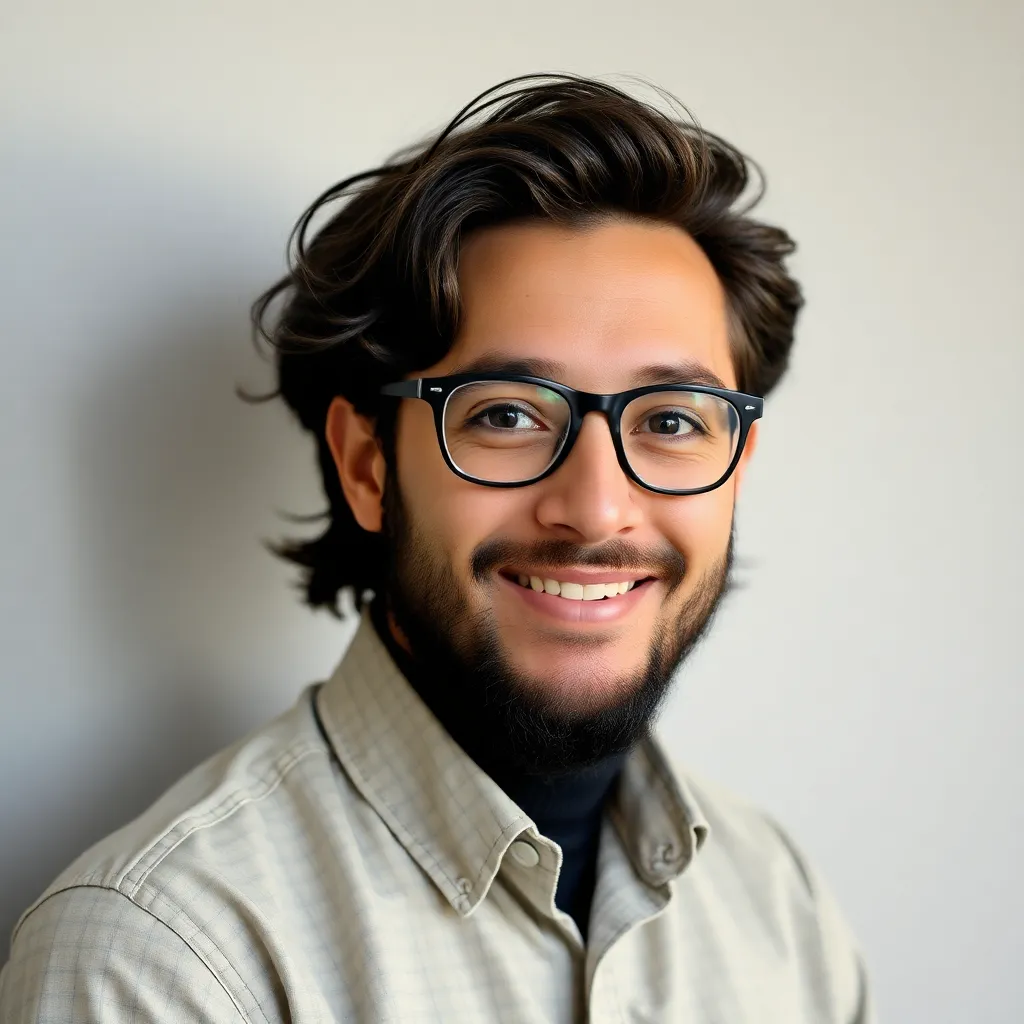
adminse
Apr 03, 2025 · 8 min read
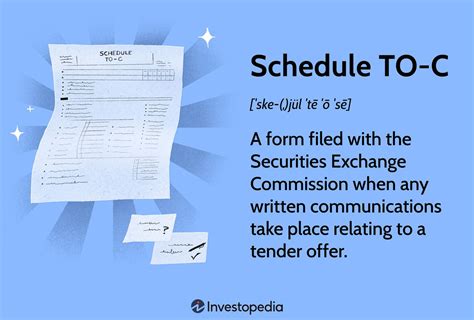
Table of Contents
Unveiling the Mysteries of schedule
in C: A Deep Dive into Time Management
What makes schedule
a game-changer in today’s landscape?
Efficient task scheduling is the backbone of modern, high-performance systems, enabling parallel processing and optimized resource utilization.
Editor’s Note: This comprehensive guide to schedule
in C has been published today. It aims to demystify this crucial aspect of systems programming, providing both theoretical understanding and practical application.
Why schedule
Matters
In the world of concurrent and parallel programming, efficient task scheduling is paramount. The ability to manage multiple processes or threads, assigning them to available resources and coordinating their execution, directly impacts system performance, responsiveness, and overall stability. Whether you're building high-throughput servers, real-time embedded systems, or complex scientific simulations, understanding and mastering scheduling techniques is crucial. The concept of "scheduling" in C, however, isn't represented by a single function or keyword but rather encompasses a range of techniques and system calls that interact with the operating system's scheduler. This article will explore these crucial elements, shedding light on their interaction and offering valuable insights. Understanding these mechanisms is vital for developers aiming to create robust and efficient software applications. The absence of a direct "schedule" function in C emphasizes the crucial role of the operating system in managing processes and threads.
Overview of the Article
This article explores the complexities of task scheduling in C, focusing on the underlying operating system mechanisms and system calls that developers utilize. We will delve into process scheduling, thread scheduling, and the role of priorities and scheduling algorithms. Readers will gain a comprehensive understanding of how to indirectly influence task execution through various C functions, enabling them to create highly optimized and responsive applications. We'll also examine potential challenges and offer practical solutions for managing complex scheduling scenarios.
Research and Effort Behind the Insights
This article draws upon extensive research of operating system principles, including documentation for POSIX standards, Linux kernel internals, and various academic papers on scheduling algorithms. The insights presented are supported by practical examples and considerations for real-world applications. The information provided is intended to be accessible to developers with a foundational understanding of C programming and operating system concepts.
Key Takeaways
Key Concept | Description |
---|---|
Process Scheduling | Managing the execution of independent processes. |
Thread Scheduling | Managing the execution of threads within a single process. |
Scheduling Algorithms | Different strategies (e.g., FIFO, Round Robin, Priority-based) used by the OS to select processes/threads for execution. |
System Calls (e.g., fork , exec , pthread_create ) |
C functions that interact with the OS scheduler. |
Process Priorities | Assigning relative importance to processes, influencing their scheduling. |
Real-time Scheduling | Scheduling mechanisms for applications with strict timing requirements. |
Smooth Transition to Core Discussion
Let's delve into the core components of task scheduling in C, focusing on how developers indirectly control execution flow through system calls and an understanding of the OS scheduler's role. We will begin by exploring process scheduling, then move to thread scheduling and the nuances of real-time scheduling.
Exploring the Key Aspects of C Scheduling
-
Process Creation and Management: The foundation of process scheduling lies in the ability to create and manage processes using system calls like
fork()
andexec()
.fork()
creates a child process, duplicating the parent's memory space.exec()
replaces the current process image with a new one, loading a different executable. The operating system scheduler then manages the execution of these processes. -
Thread Management using pthreads: For concurrency within a single process, the POSIX threads (pthreads) library provides functions like
pthread_create()
to create threads andpthread_join()
to wait for their completion. The underlying scheduling of these threads is still managed by the operating system, but the programmer has more granular control over thread creation and synchronization. -
Scheduling Algorithms and Priorities: The operating system utilizes various scheduling algorithms (e.g., First-In-First-Out (FIFO), Round Robin, Shortest Job Next (SJN), Priority-based) to determine which process or thread gets CPU time. Process priorities allow developers to influence this selection, although the specific implementation varies across operating systems. Setting a higher priority increases the likelihood of a process being selected for execution sooner.
-
Synchronization and Inter-Process Communication (IPC): When multiple processes or threads interact, synchronization mechanisms are crucial to prevent race conditions and data inconsistencies. Techniques like mutexes, semaphores, and condition variables are used for coordinating access to shared resources. IPC mechanisms, such as pipes, shared memory, and message queues, enable communication and data exchange between independent processes.
-
Real-time Scheduling Considerations: In real-time systems, meeting strict deadlines is paramount. Real-time scheduling algorithms, such as Rate Monotonic Scheduling (RMS) and Earliest Deadline First (EDF), are employed to guarantee timely execution of critical tasks. These algorithms often require careful consideration of task priorities and deadlines.
Closing Insights
Effective task scheduling in C isn't directly accomplished through a single function but is a collaborative effort between the programmer and the operating system's scheduler. By understanding process and thread management, scheduling algorithms, and synchronization mechanisms, developers can build highly efficient and responsive applications. The careful selection of algorithms and appropriate use of priorities and synchronization techniques are crucial for achieving optimal performance in various scenarios, ranging from simple concurrent programs to sophisticated real-time applications.
Exploring the Connection Between Process Priorities and C Scheduling
Process priorities directly influence how the operating system's scheduler allocates CPU time. Higher priority processes are typically given preference over lower priority ones. In Linux, for example, processes are assigned nice values (a priority scale where lower values indicate higher priority). This allows for control over the relative importance of different processes within the system. The impact is significant: high-priority processes can preempt lower-priority processes, potentially affecting responsiveness and overall system behavior. However, misusing priorities can lead to starvation, where low-priority processes never get CPU time.
Further Analysis of Process Priorities
Nice Value | Priority Level | Impact | Example |
---|---|---|---|
-20 | Highest | Process gets maximum CPU time. | Critical system processes |
0 | Normal | Process gets average CPU time. | Most user applications |
19 | Lowest | Process receives minimal CPU time (may be starved). | Background tasks with low importance |
Careful consideration of process priorities is necessary to balance system responsiveness and fairness. Incorrectly assigned priorities can severely impact performance.
FAQ Section
-
Q: What is the difference between process and thread scheduling? A: Process scheduling manages the execution of independent processes, each with its own memory space. Thread scheduling manages the execution of threads within a single process, sharing the same memory space.
-
Q: How can I set process priorities in C? A: The method for setting process priorities varies across operating systems. In Linux, you can use the
setpriority()
system call (along withgetpriority()
to get the current priority). -
Q: What are the common scheduling algorithms? A: Common algorithms include FIFO, Round Robin, SJN, Priority-based, and various real-time scheduling algorithms.
-
Q: What are race conditions and how to avoid them? A: Race conditions occur when multiple threads access shared resources concurrently, leading to unpredictable results. Synchronization mechanisms (mutexes, semaphores) are crucial to avoid them.
-
Q: What is the role of the OS scheduler? A: The OS scheduler is responsible for selecting which process or thread gets CPU time based on the chosen scheduling algorithm, priorities, and system resources.
-
Q: How does real-time scheduling differ from general-purpose scheduling? A: Real-time scheduling guarantees timely execution of tasks with deadlines, whereas general-purpose scheduling prioritizes overall throughput and fairness.
Practical Tips
- Understand your OS scheduler: Familiarize yourself with your operating system's scheduling policies and algorithms.
- Use appropriate synchronization mechanisms: Employ mutexes, semaphores, etc., to prevent race conditions and ensure data consistency in concurrent programs.
- Set priorities judiciously: Only assign higher priorities to processes that genuinely require them; overuse can lead to starvation of lower-priority tasks.
- Profile your code: Use profiling tools to identify performance bottlenecks and optimize your application's scheduling behavior.
- Consider real-time capabilities (if needed): If timing constraints are crucial, explore real-time operating systems (RTOS) and scheduling algorithms.
- Use thread pools: Manage threads efficiently by using thread pools to avoid the overhead of creating and destroying threads frequently.
- Employ asynchronous I/O: Utilize asynchronous I/O operations to avoid blocking the main thread while waiting for I/O operations to complete.
- Implement proper error handling: Thoroughly handle errors during thread creation, synchronization, and inter-process communication.
Final Conclusion
Mastering scheduling in C is a journey into the heart of concurrent and parallel programming. It's not about a single function but a deeper understanding of how the operating system manages processes and threads, allowing developers to build highly optimized and responsive applications. By implementing the strategies and techniques discussed in this article, developers can significantly enhance the performance and efficiency of their C programs, navigating the complexities of concurrent execution with skill and precision. Further exploration into specific scheduling algorithms and advanced synchronization techniques will continue to refine your understanding and capabilities in this crucial domain of systems programming.
Latest Posts
Latest Posts
-
Alligator Spread Definition
Apr 04, 2025
-
Allocated Benefits Definition
Apr 04, 2025
-
What Are Otc Derivatives
Apr 04, 2025
-
Allied Lines Definition
Apr 04, 2025
-
Alliance Of American Insurers Aai Definition
Apr 04, 2025
Related Post
Thank you for visiting our website which covers about Schedule To C Definition . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.